This post is heavily based on “Writing your first Django App” article from django documentation. The only difference is, this article contains only relevant information with simpler description.
What is Model ?
A model is the single, definitive source of truth about your data. It contains the essential fields and behaviors of the data you’re storing. essentially, your database layout, with additional metadata. Read more information about model at our another post “Understanding Django application “models””
Writing Models for Poll Django Application …
In the example poll app, we’ll create two models: Question and Choice.
- A “Question” model has a question and a publication date.
- A “Choice” model has two fields: the text of the choice and a vote tally.
- Each Choice is associated with a Question.
- Each model is represented by Python class.
Edit the polls/models.py file so it looks like this:
from django.db import models
class Question(models.Model):
question_text = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published')
class Choice(models.Model):
question = models.ForeignKey(Question, on_delete=models.CASCADE)
choice_text = models.CharField(max_length=200)
votes = models.IntegerField(default=0)
Here, each model is represented by a “class” that subclasses django.db.models.Model.
Each “field” is represented by an instance of a Field class – e.g., CharField for character fields and DateTimeField for datetimes. This tells Django what type of data each field holds.
The name of each Field instance (e.g. question_text or pub_date) is the field’s name, in machine-friendly format. You’ll use this value in your Python code, and your database will use it as the column name.
Relationship between fields of two model is defined, using ForeignKey. That tells Django each Choice is related to a single Question. Django supports all the common database relationships: many-to-one, many-to-many, and one-to-one.
Now, remember the three-step guide to making model changes:
- Change your models (in models.py).
- Run “python manage.py makemigrations” to create migrations for those changes
- Run “python manage.py migrate” to apply those changes to the databas
So, as we seen above, we changed our models.py and written the model classes.. Now, we will run the second steps from above,
$ python manage.py makemigrations
Migrations for 'polls':
my_django_project/polls/migrations/0001_initial.py
- Create model Question
- Create model Choice
By running makemigrations, you’re telling Django that you’ve made some changes to your models (in this case, you’ve made new ones) and that you’d like the changes to be stored as a migration.
Migrations are how Django stores changes to your models (and thus your database schema) – they’re files on disk. You can read the migration for your new model if you like; it’s the file polls/migrations/0001_initial.py.
let’s see what SQL that migration would run. The sqlmigrate
command takes migration names and returns their SQL: (in below command number “0001” came as seen in “my_django_project/polls/migrations/0001_initial.py” after running makemigrations above)
$ python manage.py sqlmigrate polls 0001
BEGIN;
--
-- Create model Question
--
CREATE TABLE "polls_question" ("id" integer NOT NULL PRIMARY KEY AUTOINCREMENT, "question_text" varchar(200) NOT NULL, "pub_date" datetime NOT NULL);
--
-- Create model Choice
--
CREATE TABLE "polls_choice" ("id" integer NOT NULL PRIMARY KEY AUTOINCREMENT, "choice_text" varchar(200) NOT NULL, "votes" integer NOT NULL, "question_id" integer NOT NULL REFERENCES "polls_question" ("id") DEFERRABLE INITIALLY DEFERRED);
CREATE INDEX "polls_choice_question_id_c5b4b260" ON "polls_choice" ("question_id");
COMMIT;
If we look at the above commands we can observe that,
- Table names are automatically generated by combining the name of the app (
polls
) and the lowercase name of the model –question
andchoice
. e.g. “polls_question” and “polls_choice” - Primary keys (IDs) are added automatically.
- By convention, Django appends
"_id"
to the foreign key field name. e.g. “question_id” in table “polls_choice”
Now, run migrate
again to create those model tables in your database:
$ python manage.py migrate
Operations to perform:
Apply all migrations: admin, auth, contenttypes, polls, sessions
Running migrations:
Applying polls.0001_initial... OK
Remember the three-step guide to making model changes:
- Change your models (in
models.py
). - Run
python manage.py makemigrations
to create migrations for those changes - Run
python manage.py migrate
to apply those changes to the database.
Now, lets try to create first Question using admin interface, for that create the admin user login by following steps from “Creating admin login credentials for Django”
By default when you login to admin dashboard, http://127.0.0.1:8000/admin/ you will only see “Authentication and Authorisation ” options which allows you to create Groups and Users.. like below
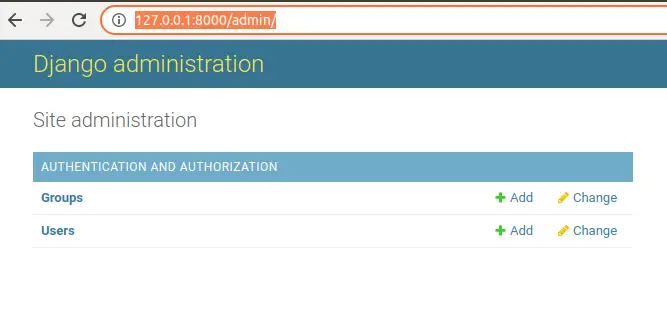
Now, to add this Model into admin dashboard, refer to next post “How to add Model to Django admin dashboard”
1 thought on “Writing first model for your Django App”