Stat() function in C is used to retrieve information about the file by accepting the filepath as an argument to it.
Stat() function can help us to extract following information about any file using C program.
- File type and mode
- Number of hard links from the file
- User ID of file owner
- Group ID of file owner
- Device ID (if special file)
- Total size, in bytes
- Time of last access
- Time of last modification
- Time of last status change
Now, for our understanding here, we will create a simple text file (helloworld.txt) and check its information using the C program.
$ vim helloworld.txt
This is the text file containing some random text for stat demo.
Now, we will write the C program as below,
$ vim file_information.c
#include <sys/types.h>
#include <sys/stat.h>
#include <time.h>
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
struct stat sb;
if (argc != 2) {
fprintf(stderr, "Usage: %s <pathname>\n", argv[0]);
exit(EXIT_FAILURE);
}
if (stat(argv[1], &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("File type: ");
switch (sb.st_mode & S_IFMT) {
case S_IFBLK: printf("block device\n"); break;
case S_IFCHR: printf("character device\n"); break;
case S_IFDIR: printf("directory\n"); break;
case S_IFIFO: printf("FIFO/pipe\n"); break;
case S_IFLNK: printf("symlink\n"); break;
case S_IFREG: printf("regular file\n"); break;
case S_IFSOCK: printf("socket\n"); break;
default: printf("unknown?\n"); break;
}
printf("I-node number: %ld\n", (long) sb.st_ino);
printf("Mode: %lo (octal)\n",
(unsigned long) sb.st_mode);
printf("Link count: %ld\n", (long) sb.st_nlink);
printf("Ownership: UID=%ld GID=%ld\n",
(long) sb.st_uid, (long) sb.st_gid);
printf("Preferred I/O block size: %ld bytes\n",
(long) sb.st_blksize);
printf("File size: %lld bytes\n",
(long long) sb.st_size);
printf("Blocks allocated: %lld\n",
(long long) sb.st_blocks);
printf("Last status change: %s", ctime(&sb.st_ctime));
printf("Last file access: %s", ctime(&sb.st_atime));
printf("Last file modification: %s", ctime(&sb.st_mtime));
exit(EXIT_SUCCESS);
}
Compile the program on Linux terminal using GCC.
$ gcc -o file_information file_information.c
$ ./file_information helloworld.txt
So, the actual command would be “./file_information your_file_name.extention ”
This command now returns the information as below,
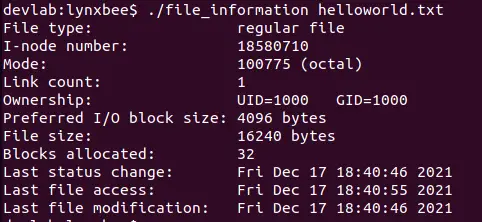