Creating a REST API using Django Rest Framework (DRF) is an essential skill for developers who want to build scalable and efficient web applications. Django Rest Framework simplifies API development by providing a robust and flexible framework for handling API requests, authentication, and serialization. In this step-by-step guide, you will learn how to set up Django Rest Framework, create your first REST API, and troubleshoot common issues.
What is Django Rest Framework?
🔹 Django Rest Framework (DRF) is a powerful, open-source framework that extends Django’s capabilities to build RESTful APIs efficiently.
🔹 It simplifies data serialization, authentication, and API views, making it easier to develop, test, and deploy web APIs.
🔹 DRF supports class-based views, permissions, and authentication mechanisms out of the box, making it a preferred choice for modern web development.
By using Django Rest Framework, developers can quickly build APIs for mobile apps, single-page applications, and backend services.
How to Set Up Django Rest Framework?
To start developing a REST API using Django Rest Framework, install Django and DRF in a virtual environment. First, create a new project and activate the virtual environment:
mkdir django_api && cd django_api
python3 -m venv env
source env/bin/activate
Install Django and DRF:
pip install django djangorestframework
Create a new Django project:
django-admin startproject myapi .
Add ‘rest_framework’ to the installed apps in settings.py
:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework',
]
Now, the Django Rest Framework is ready to be used for API development.
Creating Your First REST API with Django Rest Framework
1. Define a Django Model
To create an API, first, define a model in models.py
:
from django.db import models
class Article(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
author = models.CharField(max_length=100)
created_at = models.DateTimeField(auto_now_add=True)
Apply migrations to create the database table:
python manage.py makemigrations
python manage.py migrate
2. Create a Serializer for Data Conversion
Serializers convert model instances into JSON format, making them readable for API responses. In serializers.py
, define:
from rest_framework import serializers
from .models import Article
class ArticleSerializer(serializers.ModelSerializer):
class Meta:
model = Article
fields = '__all__'
3. Implement API Views
In views.py
, define API views using Django Rest Framework’s APIView
:
from rest_framework.response import Response
from rest_framework.views import APIView
from .models import Article
from .serializers import ArticleSerializer
class ArticleList(APIView):
def get(self, request):
articles = Article.objects.all()
serializer = ArticleSerializer(articles, many=True)
return Response(serializer.data)
4. Configure URL Routing for API Endpoints
In urls.py
, configure the API routes:
from django.urls import path
from .views import ArticleList
urlpatterns = [
path('articles/', ArticleList.as_view(), name='article-list'),
]
Now, run the Django server:
python manage.py runserver
Access the API at http://127.0.0.1:8000/articles/
to see the JSON response of stored articles.
Troubleshooting Common Issues in Django Rest Framework
1. API Endpoint Returns Empty Response
✔ Ensure that there are entries in the database:
python manage.py shell
from myapp.models import Article
Article.objects.create(title='Django API', content='Learning DRF', author='John Doe')
✔ Restart the server and check the API response again.
2. No Module Named ‘rest_framework’
✔ Confirm that DRF is installed by running:
pip show djangorestframework
✔ If not found, reinstall it using:
pip install djangorestframework
3. 403 Forbidden Error While Accessing API
✔ Ensure the Django CSRF middleware allows API requests by modifying settings.py
:
REST_FRAMEWORK = {
'DEFAULT_PERMISSION_CLASSES': [
'rest_framework.permissions.AllowAny',
],
}
✔ Restart the Django server and retry the request.
Why Use Django Rest Framework for REST API Development?
🔹 Rapid Development – Offers built-in features for quick API implementation.
🔹 Serialization Support – Converts complex data structures into JSON effortlessly.
🔹 Authentication & Permissions – Includes support for OAuth, Token Authentication, and API security.
🔹 Browsable API Interface – Provides an interactive interface for testing API responses.
🔹 Scalability & Flexibility – Suitable for small projects to enterprise-level applications.
By developing APIs using Django Rest Framework, developers can build scalable and secure web services with minimal effort.
Building a REST API using Django Rest Framework is an essential step for any modern web developer. By following this guide, you can set up DRF, create an API, and troubleshoot common issues efficiently. Whether you’re developing APIs for mobile apps, web services, or backend systems, Django Rest Framework provides the necessary tools to build robust and scalable APIs.
Start your REST API development journey today and enhance your Django web applications!
Architecture of REST API’s Development
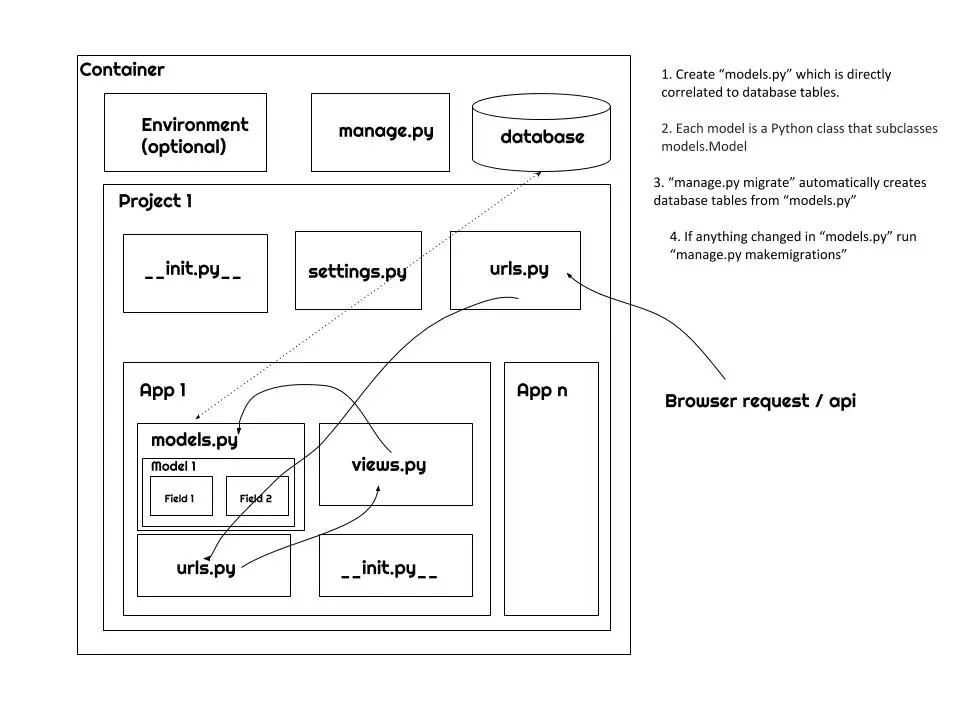
1 thought on “Beginner’s Guide: Develope Your First REST API Using Django Rest Framework”